Create and sign a transaction using the SDK and the customer backend application.
The following is the flow:
- Create a transaction through the API: At this point, transactions are created by the customer using the Create Transaction API call authenticating with the API user token and specifying the
end_user
enum as asigner_type
(see examples). The expected result is a transaction object including the simulation of effects, risks identified, and the transaction ID which can be passed to the mobile application. - Sign the transaction with the SDK: Using the transaction ID provided by Fordefi, the mobile application can invoke the signTransaction SDK method. See example code below:
async function appSignTransaction() {
try {
await signTransaction('my-transaction-id'); // transaction id to sign
} catch (e) {
const error = e as FordefiSdkErrorResult;
console.log(
`there was an error. error code :${error.code}, error message: ${error.message}`
);
}
}
import com.fordefi.fordefi.Fordefi
import com.fordefi.fordefi.FordefiError
class MainActivity: ComponentActivity() {
private
var fordefi: Fordefi ? = null
private val transactionID = "<TRANSACTION_ID>"
override fun onCreate(savedInstanceState: Bundle ? ) {
super.onCreate(savedInstanceState)
...
fordefi!!.signTransaction(transactionID) {
error: FordefiError ? ->
handleSignTransaction(transactionID, error)
}
}
private fun handleSignTransaction(transactionID: String, error: FordefiError ? ) {
if (error == null) {
Log.i("FordefiSDK",
"Trasaction $transactionID was signed successfully"
)
} else {
Log.i("FordefiSDK",
String.format(
"Failed to sign transaction with id: $transactionID. Error: %s",
error.description()
)
)
}
}
}
import FordefiSdk
class ViewController: UIViewController {
private var fordefi: Fordefi?
private var transactionID = "<TRANSACTION_ID>"
override func viewDidAppear(_ animated: Bool) {
///...
self.fordefi?.signTransaction(
transactionID: self.transactionID,
completionHandler: { error in
self.handleSignTransaction(error: error)
})
}
private func handleSignTransaction(error: FordefiError?) {
if error != nil {
print("Sign Transaction failed. Error: \(error!.errorDescription!)")
}
}
}
await fordefi.signTransaction(transactionId);
- The signed transaction will be pushed to the blockchain by Fordefi. The customer will receive webhook notifications which can be passed on to the end user.
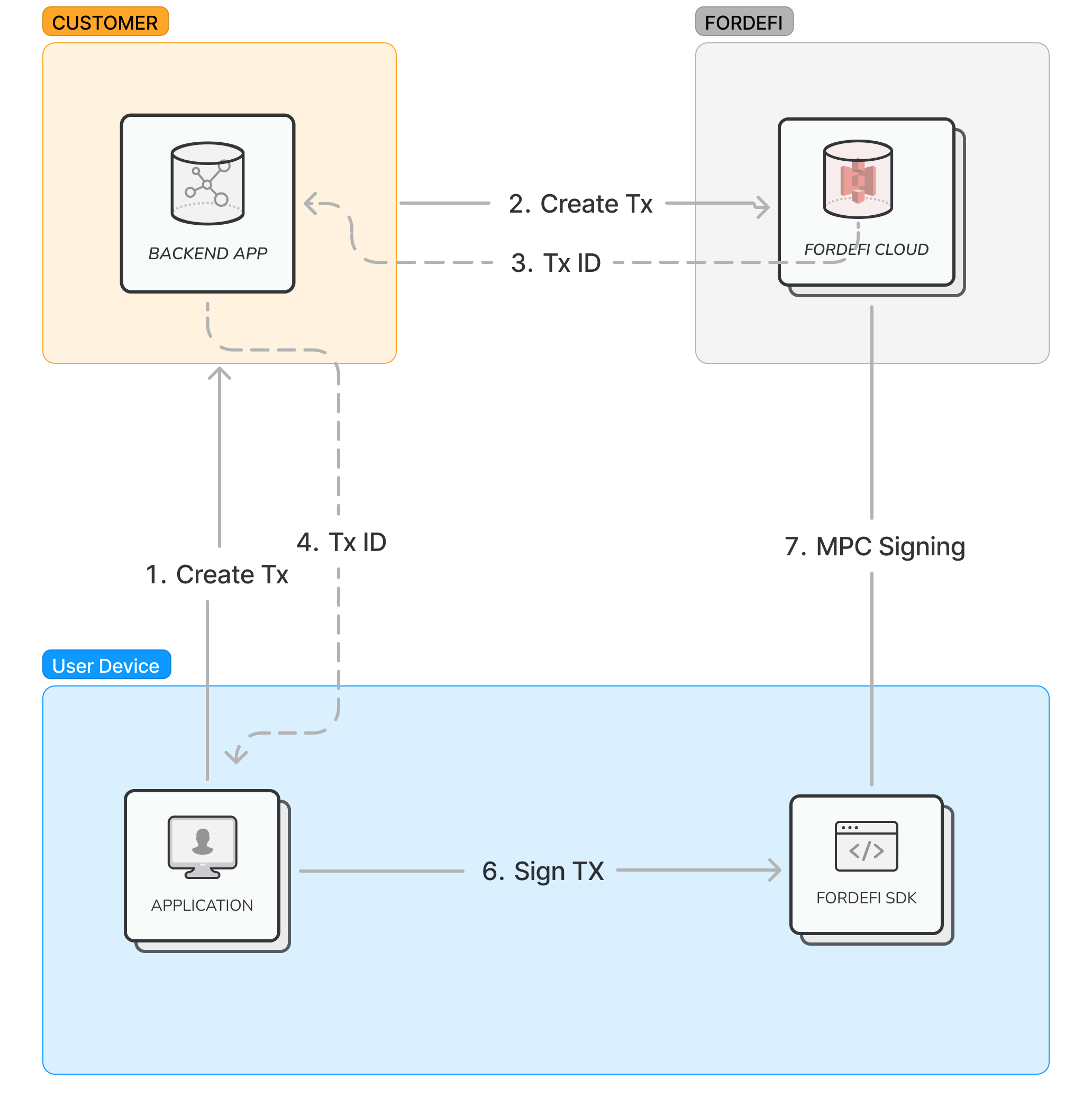