Use Webhooks
Webhooks are used to notify your application of events that have occurred in the Fordefi platform.
Configure a webhook to have a notification sent each time one of the following triggering events occurs in Fordefi:
- An incoming transaction received by the system
- A status change in an outgoing transaction (such as MINED, COMPLETED, and others)
Webhooks sent from Fordefi include partial information from a Transaction object. Call Get Transaction to receive information in full.
You can also monitor transactions using webhooks.
Monitor webhooks
In the Fordefi web console, click Settings, then click the Webhooks tab.
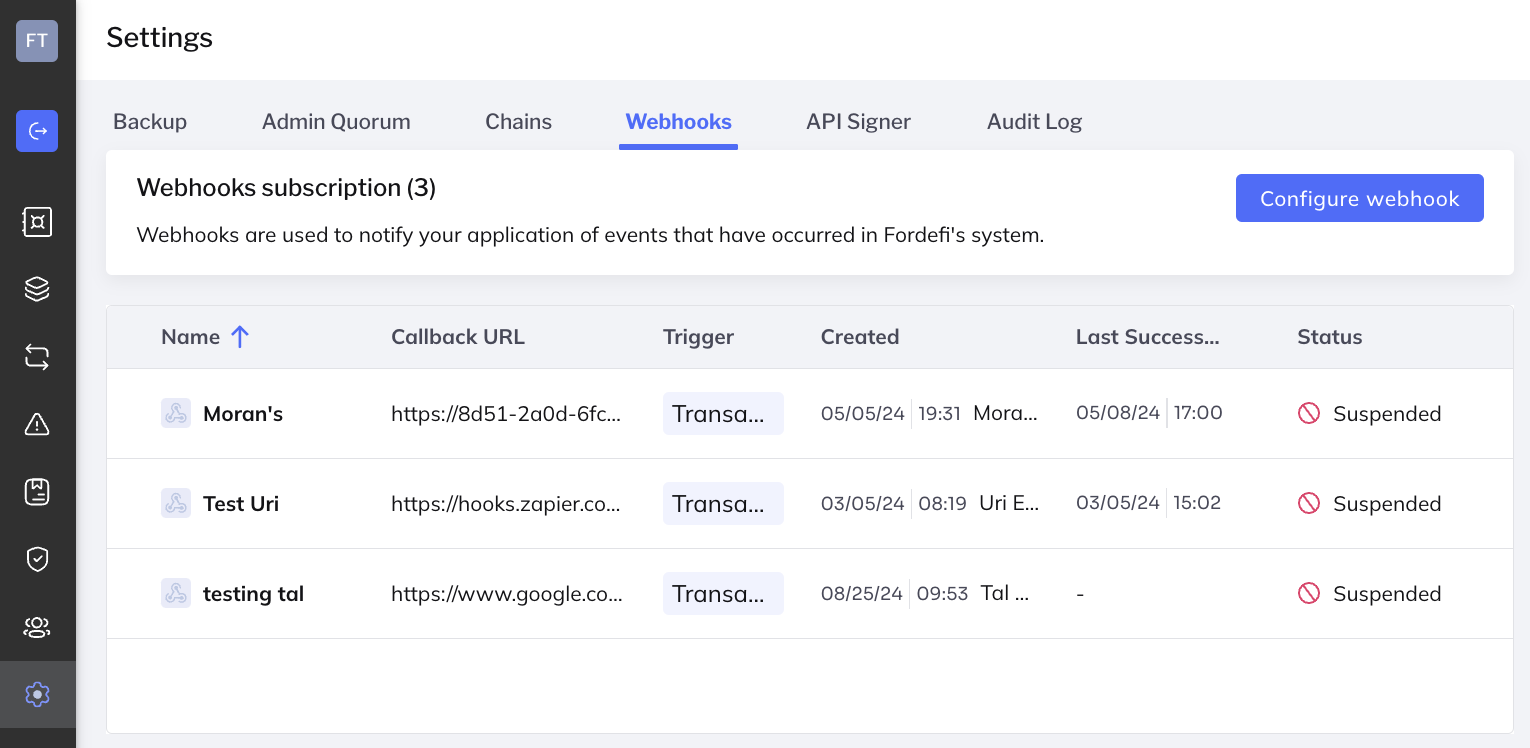
This screen displays all the webhooks you have created. The following details are shown:
Webhook name
Callback URL
Trigger (currently, transactions only)
Created date and author
Date of latest successful call
Webhook status. Can be one of the following:
Active: webhook notifications are being sent successfully.
Active with error: the last webhook was not received but the webhook will continue sending notifications.
Suspended: Fordefi tried sending notifications for five days and failed; further webhook notification was suspended. Right-click on a suspended webhook to display more options for testing, deleting, or reviving the webhook.
Configure a webhook
In the Fordefi web console, click Settings, then click the Webhooks tab.
Click Configure webhook.
In the dialog that appears, enter:
- Webhook subscription name: A descriptive name for the webhook.
- Callback URL: The URL to which Fordefi should deliver the notification. For example,
https://your-domain.com/webhook/path
. Only HTTPS addresses are permissible. - Trigger: Choose a trigger from the list. Currently, only Transactions is supported.
Click Configure.
Webhook event structure
Webhook messages sent by the Fordefi platform to your service use JSON structures. See the Fordefi API reference for more information.
Here's how webhook events are structured:
{
"webhook_id": "6b2a6f2b-2c2f-4c6b-9e1b-6d8f6a9b9c9d",
"created_at": "2025-03-11T11:45:49.530593Z",
"event_id": "6b2a6f2b-2c2f-4c6b-9e1b-6d8f6a9b9c9d",
"attempt": 1,
"sent_at": "2025-03-11T11:45:49.530593Z",
"event": {
"transaction_id": "6b2a6f2b-2c2f-4c6b-9e1b-6d8f6a9b9c9d",
"is_managed_transaction": true,
"direction": "outgoing",
"note": null,
"spam_state": "unset",
"state": "pushed_to_blockchain",
"type": "evm_transaction",
"evm_transaction_type": "native_transfer",
"hash": "0x780f21260f89d610f24eed21b34d29229611a37e0003e83dd0da91981d7131ae",
"raw_transaction": null,
"chain": {
"chain_type": "evm",
"named_chain_id": "polygon_mainnet",
"chain_id": 137,
"unique_id": "evm_137"
}
},
"event_type": "transaction_state_update"
}
Pull transaction data
Currently, webhooks contain only a small subset of all the transaction data. To obtain the full transaction data, you can extract the transaction_id
field from the webhook payload and then call the /api/v1/transactions/{id}
endpoint. Here's an example of how you can achieve this:
transaction_id = data.get("event", {}).get("transaction_id")
transaction_data = None
if transaction_id:
print("Transaction ID:", transaction_id)
fordefi_url = f"https://api.fordefi.com/api/v1/transactions/{transaction_id}"
headers = {"Authorization": f"Bearer {FORDEFI_API_USER_TOKEN}"}
try:
response = requests.get(fordefi_url, headers=headers)
response.raise_for_status()
transaction_data = response.json()
print("Transaction data:", json.dumps(transaction_data, indent=2))
except requests.exceptions.RequestException as e:
print(f"Error fetching transaction data: {e}")
else:
print("transaction_id field not found in the event data.")
Validate a webhook
Each webhook notification includes a signature on the payload. This signature is used to validate the webhook message by verifying that it actually came from Fordefi's backend and was not tampered with by any MITM attacks or malicious actors.
The following is an example of code that validates the webhook signature:
import base64
import hashlib
import ecdsa
from ecdsa.util import sigdecode_der
from flask import Flask, request
from http import HTTPStatus
app = Flask(__name__)
FORDEFI_PUBLIC_KEY = """
-----BEGIN PUBLIC KEY-----
MFkwEwYHKoZIzj0CAQYIKoZIzj0DAQcDQgAEQJ0NeDYQqqeCvgDofFsgtgaxk+dx
ybi63YGJwHz8Ebx7YQrmwNWnW3bG65E8wGHqZECjuaK2GKHbZx1EV2ws9A==
-----END PUBLIC KEY-----
"""
signature_pub_key = ecdsa.VerifyingKey.from_pem(FORDEFI_PUBLIC_KEY)
@app.route("/", methods=["POST"])
def handle():
signature = request.headers.get("X-Signature")
if signature is None:
return "Missing signature", HTTPStatus.UNAUTHORIZED
if not signature_pub_key.verify(
signature=base64.b64decode(signature),
data=request.get_data(),
hashfunc=hashlib.sha256,
sigdecode=sigdecode_der,
):
return "Invalid signature", HTTPStatus.UNAUTHORIZED
print(f"Received event: {request.get_data().decode()}")
return "OK", HTTPStatus.OK
if __name__ == "__main__":
app.run(port=8080, debug=True)
Resend notifications
Resend all failed webhook notifications
To achieve high reliability and deliverability of event notifications, Fordefi implements a webhook retry when an attempt to send a webhook message has failed. The mechanism employs exponential backoff, where the wait time is increased exponentially after every failure by two seconds, four seconds, eight seconds and so on, until the maximum backoff time of 12 hours is reached. Then, a retry is attempted each 12 hours, until three days after the first attempt, when retries end.
Resend missed notifications for a specific transaction
In the event that you missed a webhook, you can POST a Trigger Transaction Webhook call. It will resend two transaction-state-change events: one, for the first state of the transaction and a second, for the last state of the transaction.