Onboard a new user, generate keys, and create a Fordefi vault address for them.
There are two phases for onboarding new users:
- Create a new end user and receive a valid authorization token
- Generate keys and vaults
Create a new end user and receive a valid authorization token
First, create a new end user, receive a valid authorization token, then use it to log in.
Create a new end user
Organization backup
Ensure that before you add a new end user, you have performed an organization backup. Learn more. You might be blocked from adding new users if you did not back up.
Developers must use the Create End User API call from their BE application using a valid API User access token (not to be confused with the end user Authorization token). The customer is required to pass in external_id
, an argument of the Create End User call, which will be stored within Fordefi and can be used to identify the end user. Once the end user is created, it should appear also in the end user screen in the web console.
Create a new authorization token
Certain operations (such as key generation and signing) require the end user's mobile device to authenticate directly to Fordefi. Each end user has one or more unique authorization tokens. These tokens are only valid for 24 hours and it is the customer's responsibility to create new tokens once they expire and pass them to the end user's mobile application. To create a new authorization token use the Issue Authorization Token API call.
Log in to the SDK
Once the valid token is provided to the application, it can use the login method in the SDK.
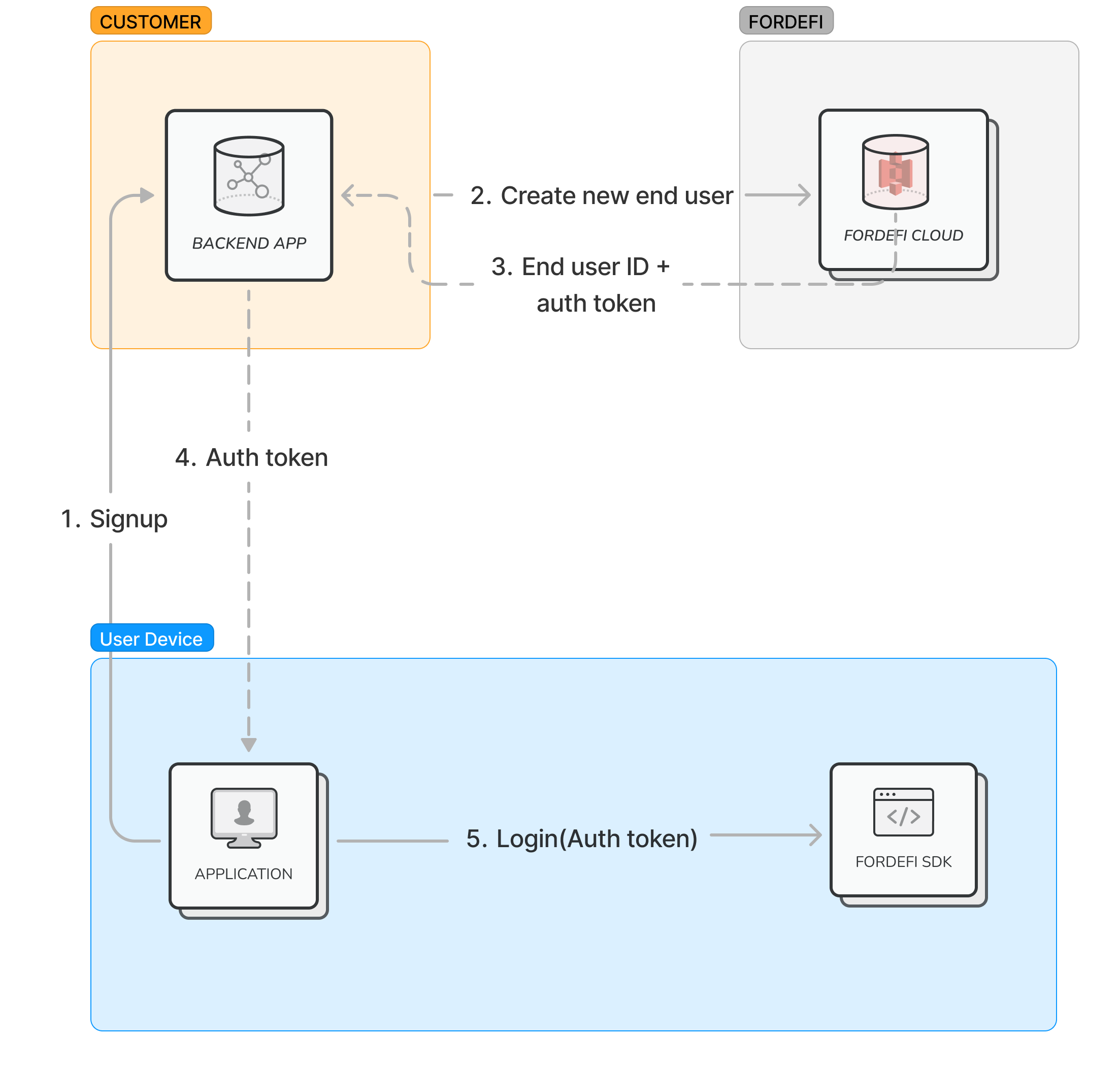
Generate keys and vaults
First, create a user keyset. Then, back up the end user's device keys and finally, create vaults for them.
Create a keyset
Now, create a Fordefi vault address for the new user.
For each end user, the platform will need to create a keyset in Fordefi's backend using the Create Keyset API call.
Once the keyset object has been created, it is passed into the generateKeys method in the mobile app to generate the different signing keys (ECDSA, EdDSA, Stark) using the MPC protocol. See the example that follows:
async function sampleapp_generateKeys() {
try {
await generateKeys('my-keyset-id', ['ecdsa']); // can be an array including ecdsa, stark, eddsa
} catch (e) {
const error = e as FordefiSdkErrorResult;
console.log(
`there was an error. error code :${error.code}, error message: ${error.message}`
);
}
}
import com.fordefi.fordefi.Fordefi
import com.fordefi.fordefi.FordefiError
import com.fordefi.fordefi.FordefiKeyType
class MainActivity: ComponentActivity() {
private
var fordefi: Fordefi ? = null
private val keysetID = "<KEYSET_ID>"
private val keyTypes = setOf(FordefiKeyType.EDDSA, FordefiKeyType.ECDSA, FordefiKeyType.STARK)
override fun onCreate(savedInstanceState: Bundle ? ) {
super.onCreate(savedInstanceState)
...
fordefi!!.generateKeyset(keysetID, keyTypes) {
error: FordefiError ? ->
handleGenerateKeyset(keysetID, error)
}
}
private fun handleGenerateKeyset(keysetID: String, error: FordefiError ? ) {
if (error == null) {
Log.i("FordefiSDK", "Keyset $keysetID was generated successfully")
} else {
Log.i("FordefiSDK",
String.format("Failed to generate keyset $keysetID: %s", error.description())
)
}
}
}
import FordefiSdk
class ViewController: UIViewController {
private var fordefi: Fordefi?
private let keysetID = "<KEYSET_ID>"
override func viewDidAppear(_ animated: Bool) {
...
self.fordefi!.generateKeyset(keysetID: self.keysetID, keyTypes: null, completionHandler: { error in
self.handleGenerateKeyset(keysetID: self.keysetID, error: error)
})
}
private func handleGenerateKeyset(keysetID: String, error: FordefiError?) {
if error != nil {
print("Generate keyset failed. Error: \(error!.errorDescription!)")
return
}
}
}
await fordefi.generateKeyset(
keysetId,
new Set([FordefiKeyType.ECDSA]),
);
To list the existing keyset and keys on a given device use the listAllDeviceKeysets method. See code sample below:
async function getKeysetsFromDevice() {
try {
const list = await listAllDeviceKeysets();
console.log(list); // ['first-keyset-id', 'second-keyset-id']
} catch (e) {
const error = e as FordefiSdkErrorResult;
console.log(
`there was an error. error code :${error.code}, error message: ${error.message}`
);
}
}
import com.fordefi.fordefi.Fordefi
import com.fordefi.fordefi.FordefiError
class MainActivity: ComponentActivity() {
private
var fordefi: Fordefi ? = null
override fun onCreate(savedInstanceState: Bundle ? ) {
super.onCreate(savedInstanceState)
...
fordefi!!.listAllKeysets() {
keysets,
error ->
handleListKeysets(keysets, error)
}
}
private fun handleListKeysets(keysets: Set < String > ? , error : FordefiError ? ) {
if (error == null) {
Log.i("FordefiSDK", "Keyset $keysetID was backup successfully")
} else {
Log.i("FordefiSDK",
String.format("Failed to backup keyset $keysetID: %s", error.description())
)
}
}
}
import FordefiSdk
class ViewController: UIViewController {
private var fordefi: Fordefi?
override func viewDidAppear(_ animated: Bool) {
///...
self.fordefi!.listAllKeysets(completionHandler: { keysets, error in
self.handleListAllKeysets(keysets: keysets, error: error)
})
}
private func handleListAllKeysets(keysets: Set<String>?, error: FordefiError?) {
if error != nil {
print("ListAllKeysets failed. Error: \(error!.errorDescription!)")
return
}
print("Available keysets:")
for key in keysets! {
print("Key: \(key)")
}
}
}
Self-custody backup
Ensure that before you create a user vault, you have backed up a mobile device's key shares. Learn more.
Create vaults
After a keyset has been created and the keys generated, a vault with a valid blockchain address can be created for the end user by the platform, using the standard Create Vault API call with the keyset ID that was generated beforehand.
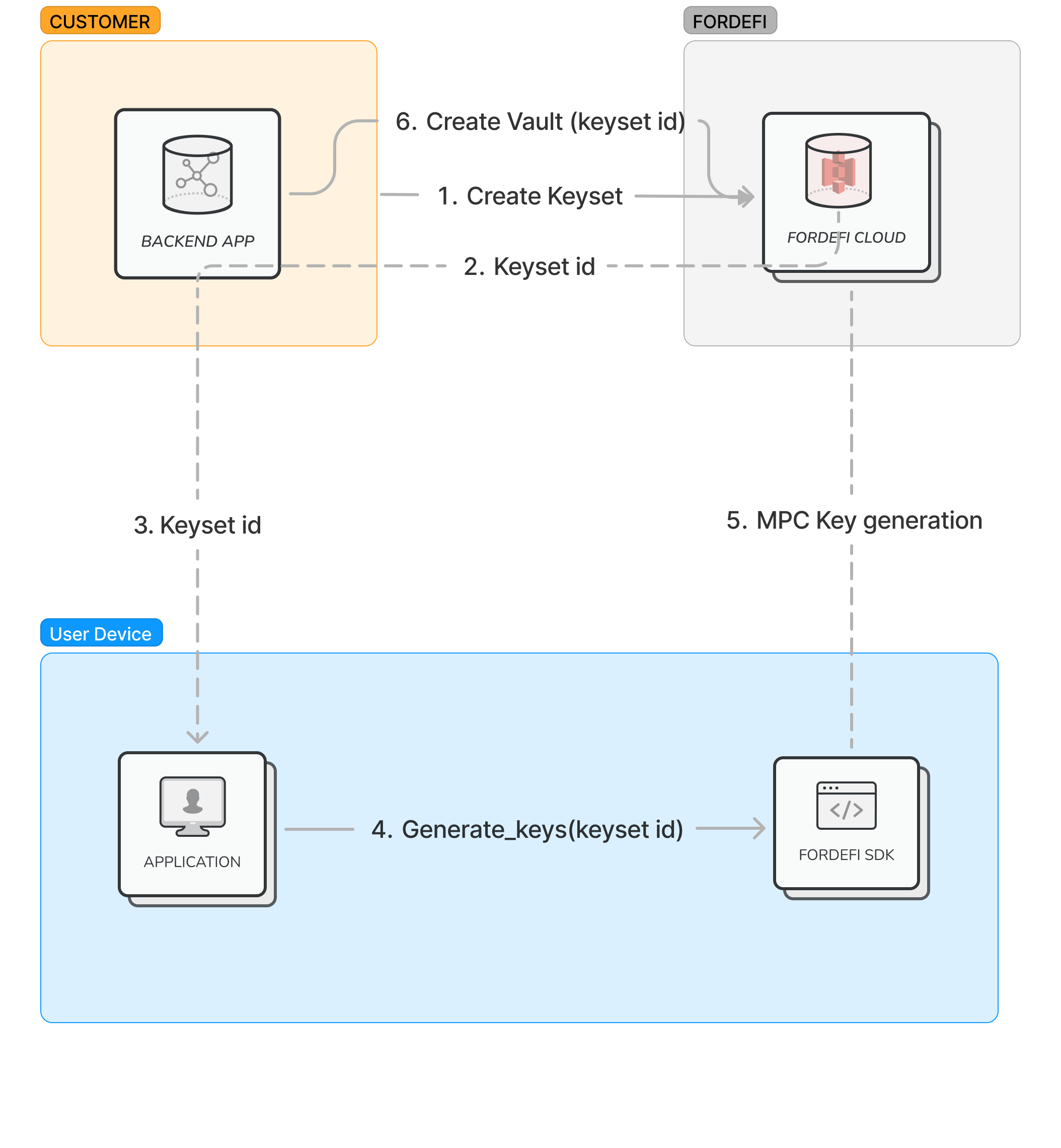